Angular: building basic calculator
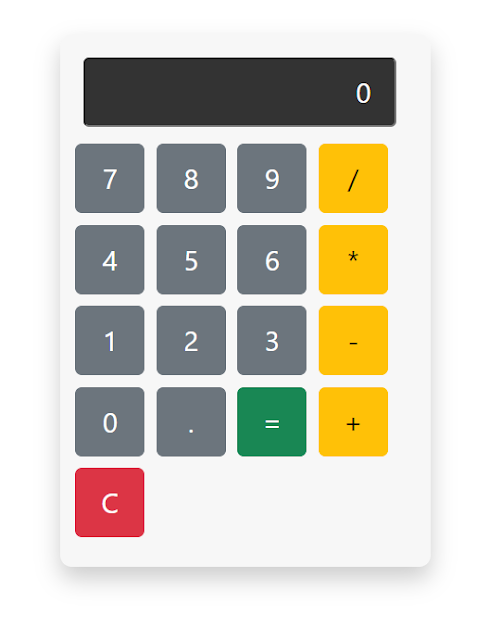
Introduction In this tutorial, we will walk through the steps to create a simple calculator application using Angular. This exercise is designed to help you practice your Angular skills and understand the basics of building a user interface and handling events. Prerequisites Before you begin, ensure you have the following: Basic understanding of Angular Angular CLI installed Node.js and npm installed Step 1: Setting Up the Project First, let's create a new Angular project. Open your terminal and run: ng new BasicCalculator cd BasicCalculator ng serve This will set up a new Angular project and start the development server. You can view the default application by navigating to http://localhost:4200 in your browser. Step 2: Designing the Calculator UI In app.component.html, design the UI of the calculator. Here's a simple structure to get you started: <div class="container"> <div class="calculator"> <div><input class=...